What is REST API?
A REST API, also known as a RESTful API or Web API, is an Application Programming Interface designed in accordance with the principles of the Representational State Transfer (REST) architectural style. This type of API offers a streamlined and adaptable approach to facilitate the integration of applications and establish connections between components within microservices architectures.
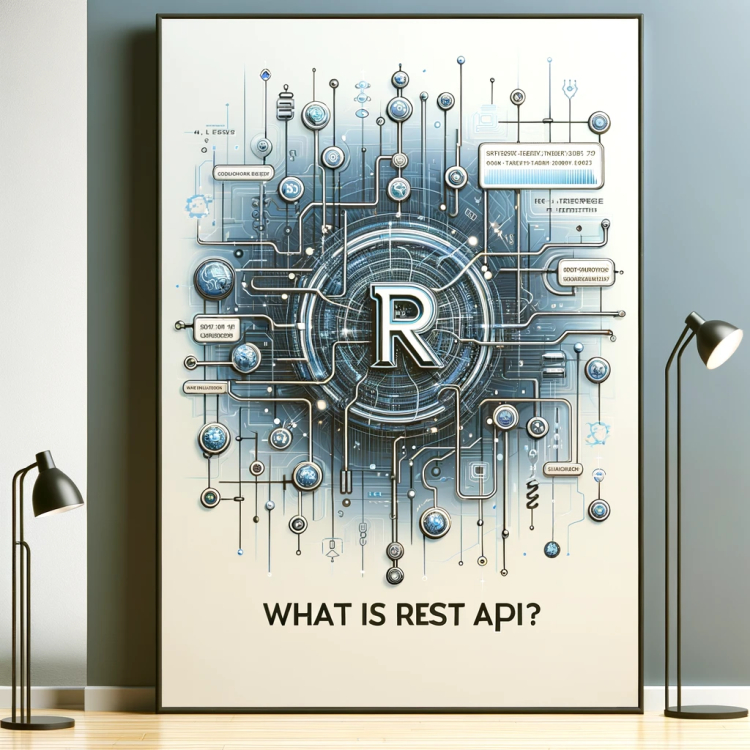
A REST API, also known as a RESTful API or Web API, is an Application Programming Interface designed in accordance with the principles of the Representational State Transfer (REST) architectural style. This type of API offers a streamlined and adaptable approach to facilitate the integration of applications and establish connections between components within microservices architectures. Its emphasis on simplicity and scalability makes it a preferred choice for developers seeking efficient communication between various software entities.
The REST architecture, or Representational State Transfer, is based on a set of principles that guide the design and implementation of web services. The key principles of REST include:
- Stateless Communication: Each request from a client to a server must contain all the information needed to understand and fulfill that request. The server should not store any information about the client's state between requests. This statelessness enhances scalability and simplifies the overall architecture.
- Client-Server Architecture: The system is divided into two separate entities – the client and the server. This separation allows for independent development, evolution, and scalability of both the client and server components. Clients are responsible for the user interface and user experience, while servers handle data storage, processing, and business logic.
- Uniform Interface: A uniform and consistent interface simplifies interactions between clients and servers. This principle is achieved through the following sub-principles:
- Resource Identification: Resources (such as data or services) are uniquely identified by URIs (Uniform Resource Identifiers). Each resource should have a stable and unique URI.
- Resource Manipulation through Representations: Resources can be manipulated and accessed using a standard set of representations, such as XML or JSON. Clients interact with representations of resources rather than the resources themselves.
- Stateless Communication: Requests from clients to servers must include all the information necessary to understand and process the request. The server, in turn, returns a representation of the requested resource or the result of the operation.
- Hypermedia as the Engine of Application State (HATEOAS): Clients interact with the application entirely through hypermedia provided dynamically by server applications. This allows the server to guide the client through available actions and transitions.
- Cacheability: Responses from the server should explicitly indicate whether they can be cached or not. Caching can improve performance by reducing the need for redundant requests.
- Layered System: The architecture can be composed of multiple layers, with each layer providing specific functionality. This modular approach enhances flexibility, scalability, and maintainability.
- Code-On-Demand (Optional): Servers can extend the functionality of a client by transferring executable code, such as scripts or applets. This principle is optional in REST and may not be used in all scenarios.
USE CASE
REST can be used with the following use cases:
- Cloud applications: The REST implementation of stateless communication is suited well for cloud applications. This is because when apps are stateless, they can be easily redeployed in the event of a failure and scaled out to accomodate workload changes
- Cloud Computing: REST supports cloud computing in controlling how URLs are decoded during client-server communication
- Microservices: REST plays a crucial role by serving as a conduit for service-to-service communication thus micoservices can work together to form a complex and functioning application.
REST API Request Structure
For the client applications and API sercers to communicate effectively, There are few things you need to put into consideration, keeping in mind that the API should determine how the client should send requests and give feedback of the information sent back to the client. The API thus acts as a middleman, defining a clear and consistent protocol for how client applications should interact with it. The components of a REST API requests are:
- Resources: In the simplest terms, a resource in a REST API is something that your application exposes or interacts with, such as data or services.
Let’s give an example in GO
package main
import (
"fmt"
)
// Task represents a resource in the API
type Task struct {
TaskID int
Description string
Status string
}
func main() {
// Example instances of Task as resources
task1 := Task{TaskID: 1, Description: "Complete project proposal", Status: "Incomplete"}
task2 := Task{TaskID: 2, Description: "Review code changes", Status: "Complete"}
// Print task information
fmt.Printf("Task 1: ID=%d, Description=%s, Status=%s\n", task1.TaskID, task1.Description, task1.Status)
fmt.Printf("Task 2: ID=%d, Description=%s, Status=%s\n", task2.TaskID, task2.Description, task2.Status)
}
In this Golang example, we define a Task
struct to represent the resource, and then we create instances (task1
and task2
) of this struct to simulate tasks. The output will display information about each task.
In a real-world scenario, you'd typically use a web framework like Gin or Echo to build a RESTful API in Golang and handle HTTP requests for creating, retrieving, updating, and deleting resources like tasks.
- Endpoints and Methods: An API endpoint is an adddess where API receives requests about a specific resource on it’s server in short a URL that provices the location of a resource on the server. A REST API can have many endpoints that correspond to an available resource.
eg. /drivers - This can be used to retrieve list of drivers with GET (HTTP method) or create a new driver with POST
Some of the HTTP methods used with endpoints include:
- GET: retrieves a resource
- POST: creates a resource
- PUT: updates an existing resource
- DELETE: removes a resource
HTTP methods correspond to CRUD Operations. For example, the HTTP methods POST, GET, PUT, and DELETE correspond to create, read, update, and delete CRUD operations.
- Parameters: Think of API parameters as a way to customize what the API sends back to you. They let you narrow down the data to get just what you want. API parameters are like filters when you're shopping online. They help you choose the right size, color, and other options, so you get the perfect results from the API.
Types of REST API Parameters
- Path Parameters
They mainly identify a specific resource within the API. They usually come after a resource name
eg.
GET /pet/{driverId}
- Query String Parameters
Filter, sort, or provide additional options for your request. They are added at the end of the URL after a question mark (?
)
- Header Parameters
Provide metadata about the request itself (not directly about the resource). Included in the HTTP headers.
common uses include:
Authentication: Sending API keys or tokens (Authorization: Bearer my_api_key
)
Content Type: Indicating the data format of the request or response (Content-Type: application/json
)
Cache Control: Instructions on how to cache responses (Cache-Control: max-age=3600
)
- Request Body Parameters
Send substantial data to the server, typically used with POST and PUT methods to create or update resources.
Its usually not in the url but within the body of the HTTP request
{
"title": "My New Article",
"content": "This is the content of my article...",
"category": "technology"
}
What's Your Reaction?
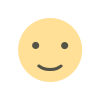
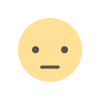
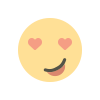
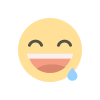
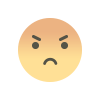
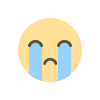
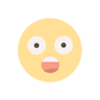