Load Balancing with Golang
Load balancing is the process of efficiently distributing incoming network traffic across a group of backend servers.
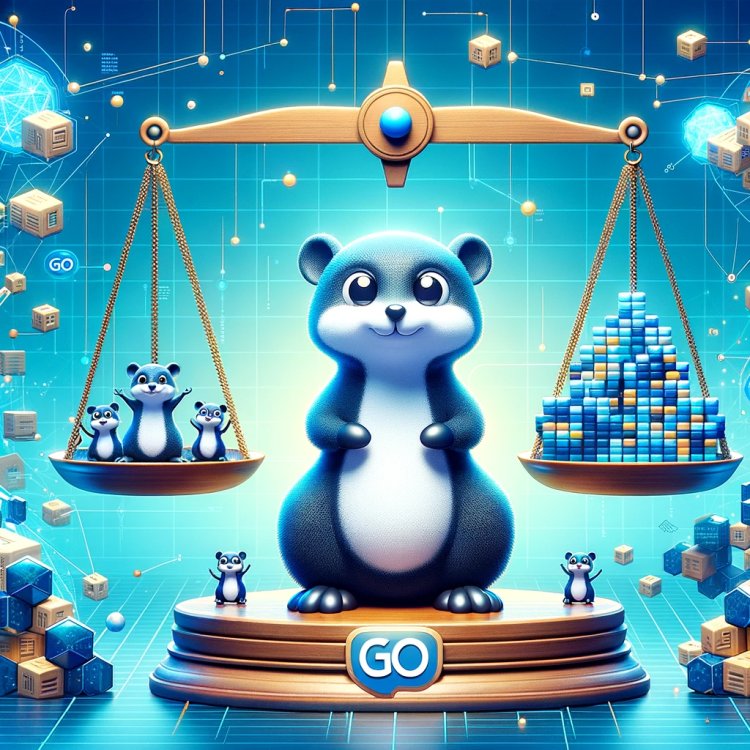
Load balancing is the process of efficiently distributing incoming network traffic across a group of backend servers.
In an instance where there are constant requests from users mostly common with banking systems etc, having a traffic ‘cop’ in between the user requests goes a long way to efficiently maximize the requests to maximize on speed and capacity utilization to ensure no server is overworked. If one server goes down, the load balancer redirects traffic to the remaining online servers.
In the above instance, when a user makes a request, it goes through the load balancer to determine which server is available.
A reverse proxy is required in this as well for security. We are using the http util package to create the reverse proxy. The reverse proxy redirects the request while hiding the address of the server
type simpleServer struct {
addr string
proxy *httputil.ReverseProxy
}
func newSimpleServer(addr string) *simpleServer {
serverUrl, err := url.Parse(addr)
handleErr(err)
return &simpleServer{
addr: addr,
proxy: httputil.NewSingleHostReverseProxy(serverUrl),
}
}
The loadbalancer will be implemented as a struct with a port, server and roundrobincount to check between the alive servers.
type LoadBalancer struct {
port string
roundRobinCount int
servers []Server
}
func NewLoadBalancer(port string, servers []Server) *LoadBalancer {
return &LoadBalancer{
port: port,
roundRobinCount: 0,
servers: servers,
}
}
We also create a function to create a new loadbalancer and server based on the struct.
We have 2 methods
isAlive method checks if the server is up or not and
Get address method to get address of the server
func (s *simpleServer) Address() string { return s.addr }
func (s *simpleServer) IsAlive() bool { return true }
An overview of this;
We have a main function which creates a server list and calls the server proxy function to get the next available server. Thus checks if the server is alive through roundrobin
What's Your Reaction?
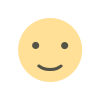
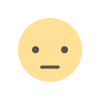
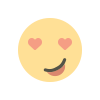
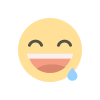
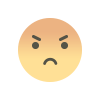
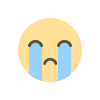
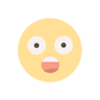