1. Introduction to golang
Introduction to Go programming language. In this guide we will be going through installing golang, writing our fisrt code, variables and data types, control Flow, Functions, packages, concurrency and Error handling
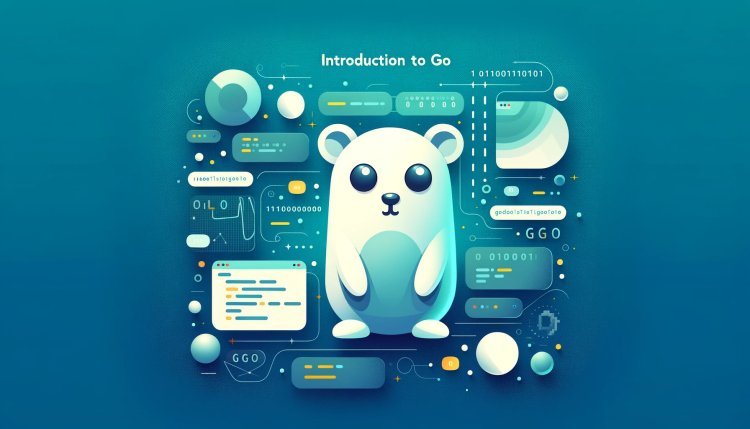
Go, commonly referred to as Golang, is a statically typed, compiled programming language designed for simplicity, efficiency, and ease of use. Developed by Google, Go has gained popularity for its clean syntax, strong support for concurrency, and efficient compilation. This tutorial aims to provide a gentle introduction to Go for beginners. I will be taking you through the process step by step and build simple projects for ease of learning. Feel free to comment if and ask for clarification. I will be introducing videos along the way for visual learners. I hope this will help any beginner struggling to understand golang.
1.1 Installing Go
Windows
Installing golang is pretty easy, visit the official Go website and dowload the installer for windows. Follow the steps and you will have go installed in your system.
After that you will need to go to the environment variables and add the go path in the environment variable. If you open the terminal and type: go version
it should show the currently installed.
Linux
I will be using linux for the entire of the tutorial. If you don't want to tamper with your windows system and would like to use linux. You can just install windows subsystem for linux, should work just fine as all you need is the terminal.
Installing on linux is pretty straight forward. Open your terminal and type:
sudo apt update
sudo apt upgrade
sudo apt install golang-go
If you type go version
, it should show the currently install golang version
1.2. Writing your first code
For ease of development, since we will be making a lot of beginner friendly projects, I'll show you how I usually set up my environment. My IDE choice is visual studio code
You simply need to open vscode, navigate to extensions and install Remote-SSH. This will make it easy for you to access your code directory. When you click on the remote-ssh button on the bottom left, it will prompt you to log into your server.
Next step is to create a directory where you will be running your go projects. In my case, I created a main Directory: GOLANG with a sub-directory of the current topic; Introduction to golang
As the culture dictates. We have to begin by writing hello world program to introduce you to the syntax. I have created a file; hello.go. Add the following code and save
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
open a terminal withing vscode and run go run hello.go
As you can see. the output is shown on the terminal. To break down what this means:
package main: A package is a way to group functions and is made up of files in the same directory. The main package therefore instructs the go compiler to compile the code as an executable program instead of a shared library.
import "fmt": the fmt package implements formatted input/output. In our case we want to output Hello, World!. We therefore import the fmt package
func main: Finally we declare the main function using the keyword func. The function call; fmt.Println("Hello, World!") will call the Println function from the fmt package to therefore print the line to the standard output, in our case printing Hello, World! on the console.
What's Your Reaction?
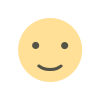
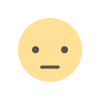
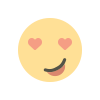
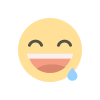
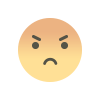
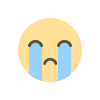
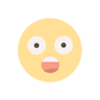